Tools For Your Tool-Belt
As a freelance developer its good to have a number of different tools in your tool-belt for when the situation demands. Firebase, and in particular, Firebase Authentication, has proven really useful over the past couple of years.
JWT
Most projects that I work on nowadays require some sort of user authentication. In the past I have used JWT or basic auth as my go-to solutions for authentication. However, even those these methods are fairly straightforward, I still had the nagging feeling that “This is a common problem, there must be a way to make this even easier.”.
JWT is great and easy to get started with but it is not always plain sailing; basic auth is well….. basic. JWT is very similar to Firebase Authentication in that you can use a 3rd party to authenticate users (using the Auth0 service) and not have to set up your own authentication server and process. However, manually persisting tokens on the client side and handling the clean up on expiry, logout etc, still felt verbose to a lazy dev like myself.
Firebase Auth
Firebase Authentication is easy to set up, the wide range of SDKs are easy to use, is free to start using, and “supports authentication using passwords, phone numbers, popular federated identity providers like Google, Facebook and Twitter, and more”.
Most of my experience using Firebase authentication has been in web apps or hybrid mobile app development; below are some really quick steps on how to get started for web:
- Sign up for a Firebase account and create a new project.
- Choose “Add firebase to your web app” and follow the instructions i.e. include the JS library and add the configuration details to your app.
- Add a screen which asks the user for an email address and password. Then pass these details to the “createUserWithEmailAndPassword” SDK function to create a new user.
- Add another screen which asks for an email address and password, then pass these details to the “signInWithEmailAndPassword” function. When the function returns you should now have an authenticated user! I could go into more detail on the other methods you can use e.g. “signOut”, but most of them really are self-explanatory and the docs do a great job of showing you how.
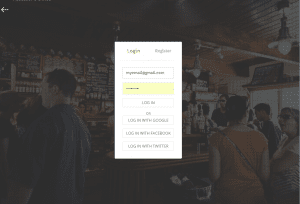
Add a login screen with text boxes and a login/register button, then hook the button click to the Firebase SDK methods
Facebook, Google, Twitter, GitHub
To add Facebook, Google, Twitter or Github authentication just:
- Enable the required type of authentication through the “Authentication” tab on your Firebase console (following any specific instructions for each provider).
- Add a button to your login page for each provider you wish to use.
- Hook up the button click to use the appropriate SDK method on the client e.g. “signInWithPopup(new firebase.auth.GoogleAuthProvider())”
- After the user has authenticated themselves through the popup window, your call should return and you should now have a [Google] authenticated user.
- *A word of caution for hybrid mobile developers* Popups will not work on your hybrid mobile app, you will need to use native Facebook/Google libraries to achieve the same thing e.g. using facebook sign-in for Ionic.